As a software engineer based in Dublin, Ireland, I often find myself juggling a busy schedule. Managing my fitness classes, work meetings, and personal commitments can be quite a challenge. To streamline this process, I decided to embark on a project to automatically create Google Calendar events from Gympass confirmation emails. In this blog post, I’ll walk you through the steps and code I used to accomplish this task.
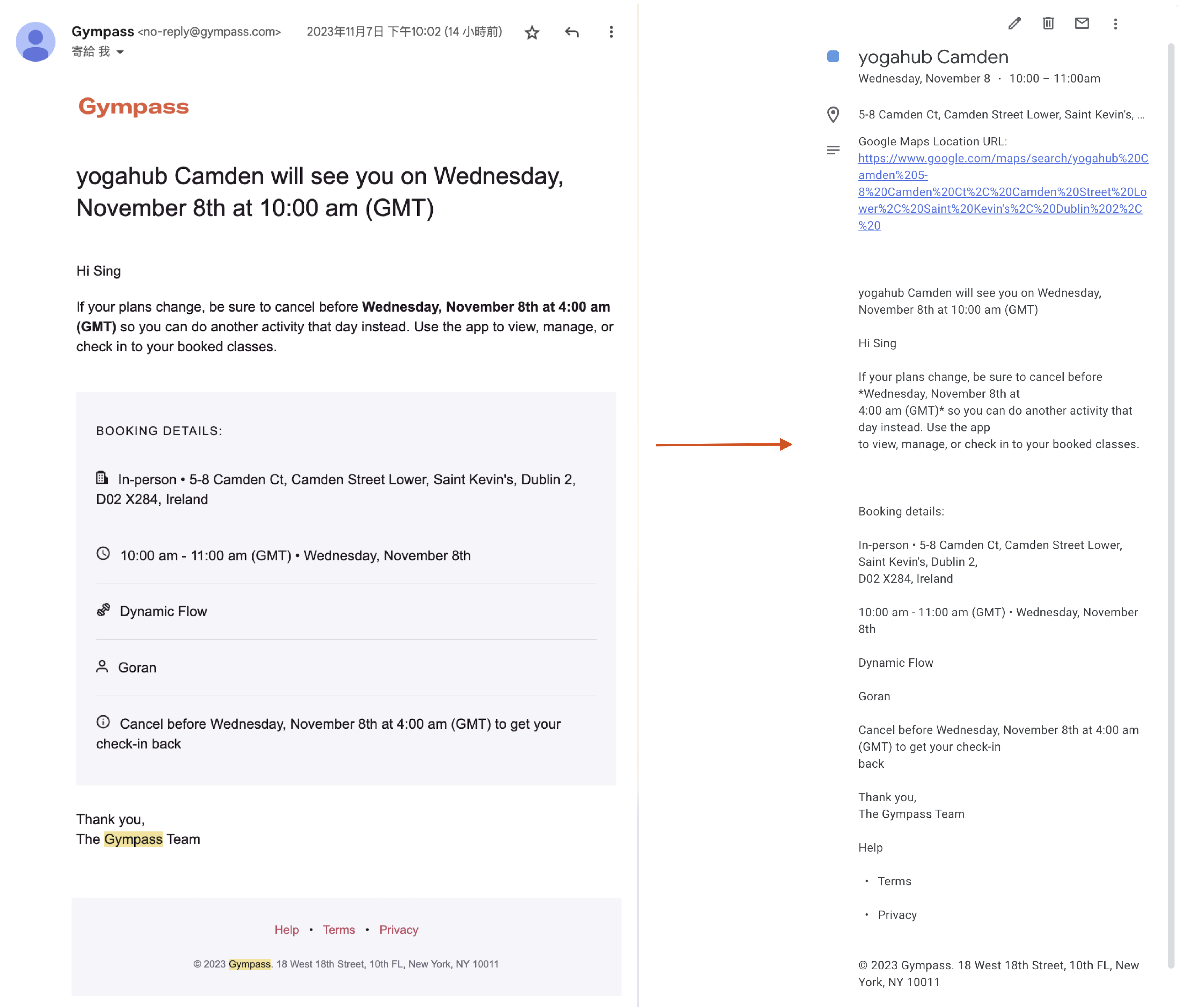
The Problem
Gympass, a popular fitness service, sends confirmation emails whenever I book a fitness class. These emails contain valuable information about the class, such as the date, time, location, and instructor. Manually adding these details to my Google Calendar was becoming a time-consuming task.
The Solution
To automate this process, I created a Google Apps Script that runs in my Gmail account. This script scans my inbox for Gympass confirmation emails, extracts the relevant information, and creates corresponding Google Calendar events. Here’s an overview of how it works:
Create a Google Apps Script Project:
- Open your Gmail account in a web browser.
- Click on the “Apps Script” icon in the top-right corner (it looks like a square with a pencil).
- This will open the Google Apps Script editor.
Write the Script:
- In the Google Apps Script editor, copy and paste the script code provided in this blog post.
Save and Name the Project:
- Give your project a name by clicking on “Untitled Project” at the top left and entering a name.
Authorization:
- The script will need authorization to access your Gmail and Google Calendar. Click on the run button (▶️) in the script editor.
- Follow the prompts to grant the necessary permissions to the script.
Trigger the Script:
- To automate the script’s execution, you can set up triggers. Click on the clock icon ⏰ in the script editor.
- Create a new trigger that specifies when and how often the script should run. You might want it to run periodically, such as every hour.
Email Search: The script starts by searching your inbox for emails from `[email protected]`. This ensures that it only processes Gympass emails.
Email Parsing: For each Gympass email found, the script extracts the email’s subject and plain text body.
Cancellation Handling: If the email subject indicates that you canceled a booking, the script performs a cancellation action (you can customize this based on your business logic).
Data Extraction: For non-cancellation emails, the script removes any unnecessary footer text and URLs from the email body.
Event Details Extraction: Using regular expressions, the script extracts the date, time, location, and event title from the cleaned email body.
Month-to-Number Conversion: It converts the month name to a numeric value for creating a
Date
object.Event Creation: With all the details in hand, the script creates a new Google Calendar event. It includes the event title, start and end times, location, and a Google Maps link to the event’s location.
Duplicate Event Check: Before creating an event, the script checks if an event with the same date, time, title, and location already exists in the calendar to avoid duplicates.
Code Organization
To keep the code clean and maintainable, I divided it into several functions:
extractAndCreateCalendarEvent
: The main function that orchestrates the entire process.extractEventDetails
: Extracts event details from the email body using regular expressions.monthToNumber
: Converts month names to numeric values.createCalendarEvent
: Creates a new Google Calendar event.isEventExist
: Checks if an event with the same details already exists.removeFooterAndUrls
: Removes unnecessary footer text and URLs from the email body.cancelEvent
: Placeholder for handling event cancellations (customize based on your needs).parseTime
: Parses time in HH:mm a format and returns it as milliseconds.
1 | // Main function to process emails and create calendar events |
Conclusion
Automating the creation of Google Calendar events from Gympass emails has significantly reduced the time and effort required to manage fitness classes and appointments. With this script running in the background, you can focus on your workouts and let technology take care of the scheduling.
By following the steps outlined in this blog post, you can set up your own automated email-to-calendar integration and adapt it to your specific needs. Happy coding!